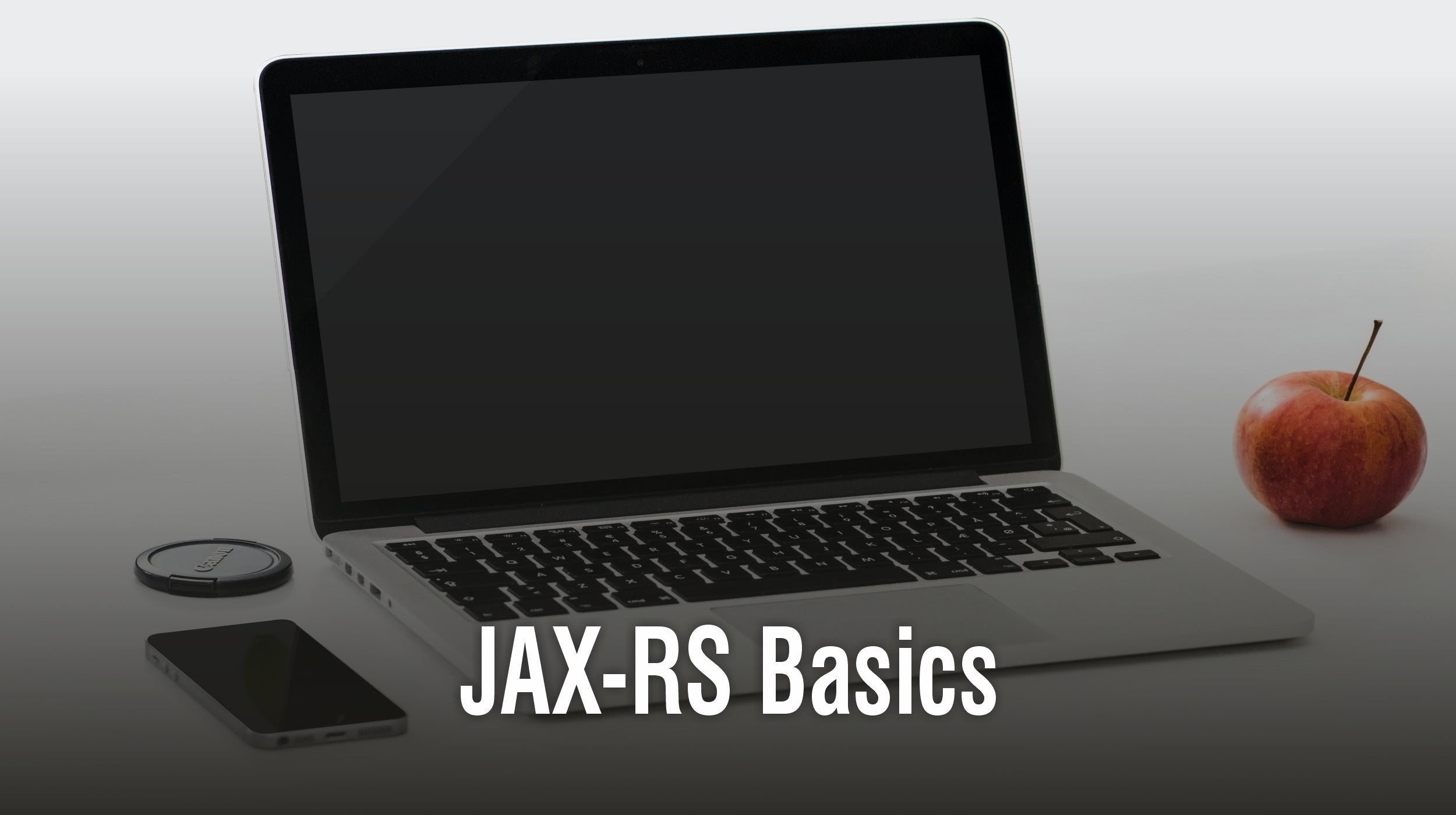
JAX-RS Basics
by Vibrant Publishers
Introduction
The implementation of REST framework in Java is done through JAX-RS API. It serves as the basic building block for RESTful services in Java. Let’s take a look at the basics of JAX-RS.
JAX-RS
JAX-RS (Java API for RESTful Web Services) is also part of the JSR, with a detailed specification definition. Starting with JAVA EE 6, this technology has been built, similar to RESTful WCF in .NET, that exposes the RESTful service by using some simple annotations in the conventional method.
The current version of JAX-RS in use is version 2.0. The below-given diagram shows the features of this version.
HTTP Methods and annotations
We know that the REST APIs work through HTTP protocol. The following are the HTTP methods used in REST via annotations.
- @GET: Defines HTTP GET request method.
- @PUT: Defines HTTP PUT request method.
- @POST: Defines the HTTP POST request method.
- @DELETE: Defines HTTP DELETE request method.
A basic GET request
Let’s see how a basic HTTP GET request is implemented using JAX-RS API using a simple ‘Hello World’ example.
@Path(“/”)
public class SampleWebService {
final String XMLNS_NAMESPACE = “http://localhost:8080/rest/service”;
final String ROOT_NODE = “root”;
@GET
@Path(“/json/hello”)
@Produces(MediaType.APPLICATION_JSON)
public JAXBElement<String> getHelloWorldJSON() {
JAXBElement<String> result = new JAXBElement<String>(new QName(“”, ROOT_NODE), String.class, sayHelloWorld());
return result;
}
……….
}
private String sayHelloWorld() {
return “Hello, this is a sample JAX-RS API!”;
}
|
- @GET indicates that the service can be accessed directly in the browser address bar (for the GET method in HTTP requests).
- @Path used here twice, the first on Class, the base address of service, and the second time on a method, representing the URL of a specific service method.
- @Produces defines the output type of method. Here we are opting for a JSON string format output.
We will also deploy the web.xml file in the root folder of the application.
Now this REST API can be accessed at the address
http://localhost:8080/rest/service/json/hello
|
This will return output in the HTML browser as
{“root”: “Hello, this is a sample JAX-RS API!”}
In this way, any resource at the server side is made available to the client through simple REST API calls over HTTP. Similarly, create, update and delete operations on a resource can be done at the server-side by calling corresponding REST API commands from the client-side.
Content Negotiation
While using REST APIs, we are sharing resources that are represented by multiple data types. JSON and XML are the two most popular content types that are commonly used.
The JAX-RS annotations @Produces, @Consumes are used to specify the content format. As the name suggests, @Produces annotation specifies the type of content produced by a method. The @Consumes annotations specify the type of content that a method is expecting as input.
For example, @Produces({“application/xml,application/json”})
means the method will produce content in two MIME types, XML and JSON.
One thing we should understand here is the differences between data and data format. Data can be anything and the data format is the representation of that data. Data transformations occur before or after transmission.
When there is an equal match with the request and response type, the server will return a 200 OK response or a 400 Bad Request if the data format is not matching with the MIME type that the server expects.
Now, let us say the client does not specify any content format, then in JAX-RS 2.0 there is a factor called ‘QS’ ie. ‘QUALITY FROM SERVER’. It can take value from 0 to 1. So in the above scenarios ‘qs’ factor instructs the server to choose a content type that is the default content format.
If we consider, @Produces({“application/json qs=0.8; ,application/xml; qs=0.75”}), it is clear that the server has to consider the JSON format.
Request Matching
Request matching is a process where the client API request is mapped to the corresponding method at the server-side. This is achieved using the @Path annotation. The value of the @Path annotation is an expression that indicates the relative URI. Consider the following example:
@Path(“/orders”)
public class OrderResource {
@GET
public String getAllOrders() {
…
}
}
|
Here if you send an HTTP request of GET /orders to the server, that would dispatch to the getAllOrders() method.
The JAX-RS has strict precedence and sorting rules for URI expressions matching. This is based on the ‘most specific match wins’ algorithm. You must also abide by the URL encoding rules that only allow alphabetic characters, numbers and some special characters only.
Summary
The JAX-RS library has specifications and rules that will help a developer to build web services in the RESTful way. In JAX-RS the importance of annotations and how HTTP methods are mapped using these annotations are important to understand. The request – response matching mechanism and specifying the content type while making a request are also important concepts in JAX-RS implementation.
Share