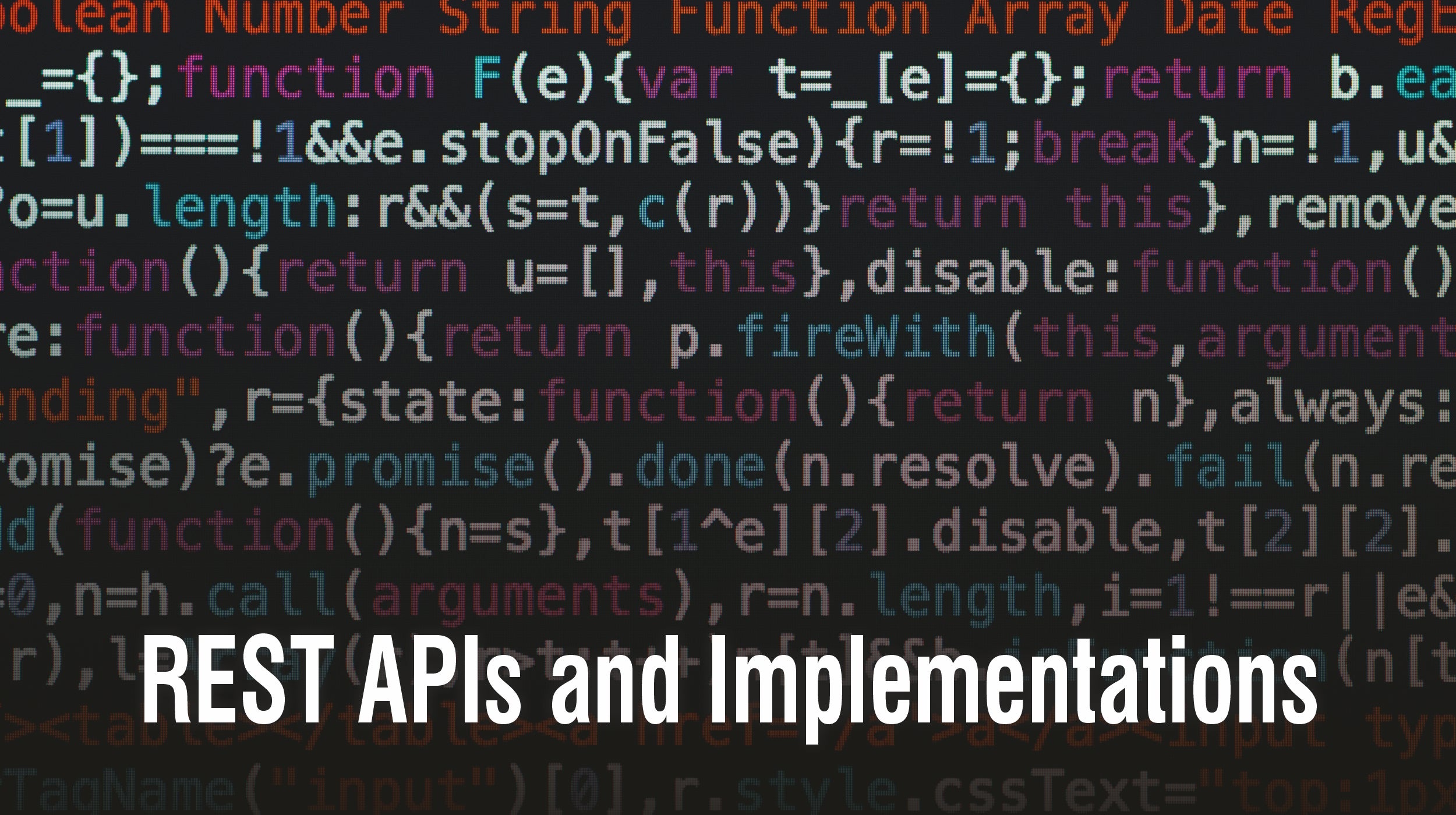
REST APIs and Implementations
by Vibrant Publishers
Introduction
Building a RESTful service is specific to every programming language. There are different libraries and implementations of REST in each programming language. In this post, we are going to look into the details of how Java language implements REST and how we can build RESTful applications in Java.
Java and REST
REST got into the Java world through the release of JavaEE 6. It included the JAX-RS API that is responsible for REST API creation using Java. JAX-RS follows the REST framework and the HATEOAS principle. The current version of JAX-RS is version 2.0.
JAX-RS uses annotations for ease of development and deployment of web services. These annotations are part of the java package javax.ws.rs. The basic annotations provided by JAX-RS are:
- @Path
- @GET, @POST, @PUT, @DELETE, @HEAD
- @Produces
- @Consumes
- @PathParam
JAX-RS Implementations
There are many implementations of JAX-RS available in Java. They show minor variations in their ways of defining and using REST APIs. But the basic procedures remain the same in all of them.
A few of those implementations are:
- Jersey: This is the reference implementation of JAX-RS from Sun Microsystems. This is one of the most widely used libraries for building RESTful services in Java.
- Spring REST: This REST library is part of the Spring web framework. If you are using the spring framework for building your application, making it restful is very easy. It follows an MVC architecture.
- RESTeasy: RESTeasy is developed by JBOSS and is part of their application server. This implementation has server-side caching and GZIP compression features.
- Apache CXF: This implementation of JAX-RS specification is by Apache. It is an open source library available for free download and modification.
- Restlet: This is again an open source implementation of JAX-RS 1.1 specification. It is a light-weight library that supports major media types and all REST specifications.
Serialization Frameworks
When we implement REST APIs depending on the request and response types, one would always need to use some kind of object serializers. The following are some of the popular serialization frameworks:
- JAXB
It stands for “Java Architecture for XML Binding”. This library helps in the conversion of java objects into an XML data format and vice versa. One can find this API in the javax.xml.bind package. There are many implementations of JAXB in the form of annotations that can be used in java code for conversions.
- JACKSON
Jackson is a JSON processor that is used widely in the Java world for converting Java objects into JSON document format and vice versa. This implementation is part of the Jersey library.
Building a RESTful Application
As you can see, there are many choices in the Java world for building a RESTful application. The libraries and implementations listed here can be used with any Java framework. It is our choice to go for a particular library based on our use case and technologies that we are adopting.
General steps for building any RESTful services would be:
- Identify resources and build URIs
- Select or build representations.
- Identify method semantics.
- Select response codes.
The below diagram shows a simple technology stack for a RESTful application.
|
Summary
After the introduction of REST in JavaEE from version 6 onwards, a lot of implementations and libraries came into existence. The JAX-RS has got strict implementation principles and guidelines for building RESTful API services. As a java web services developer it is important to understand which library to use and how to build RESTful services adhering to the REST principles.
Share