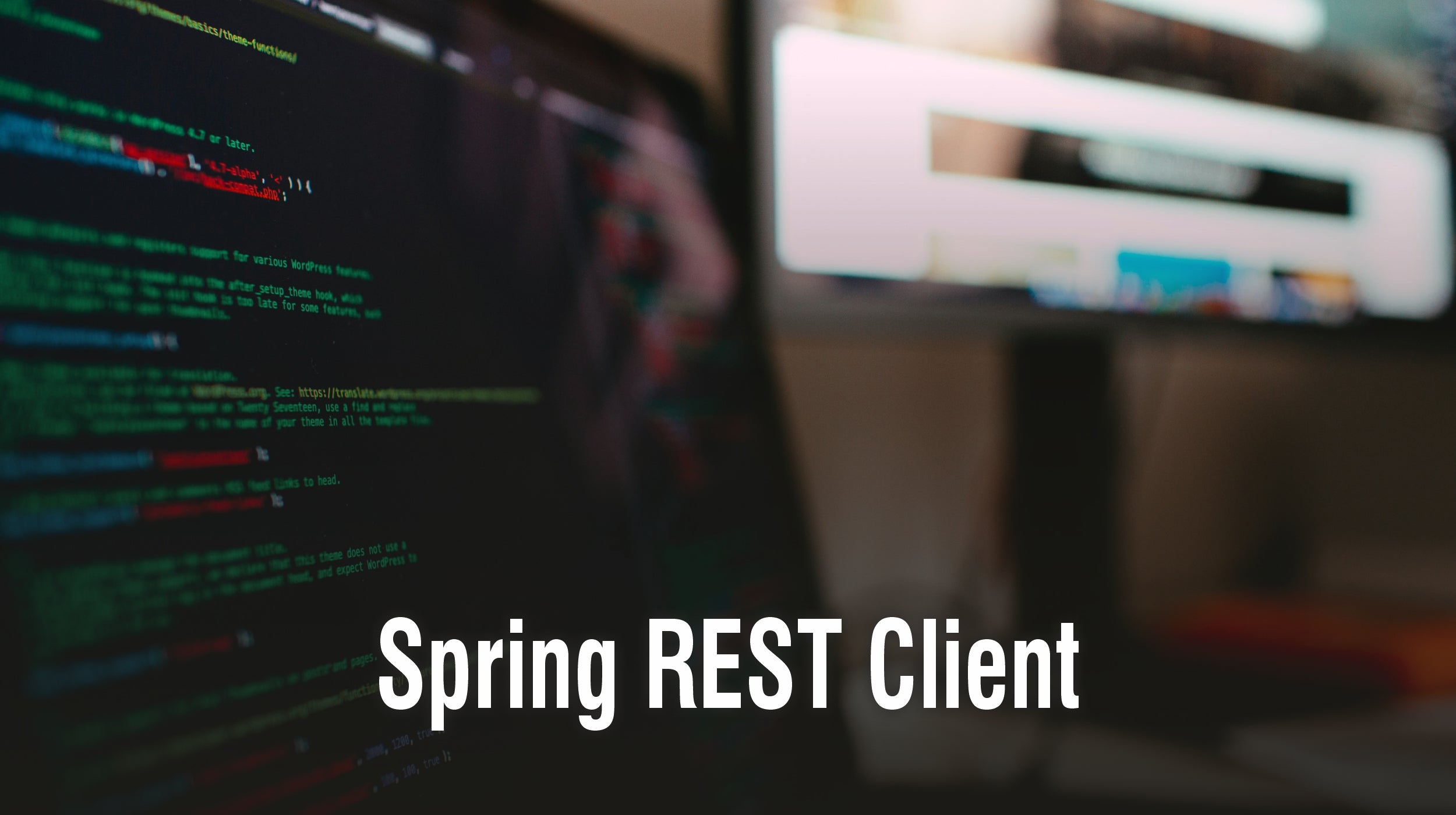
Spring REST Client
by Vibrant Publishers
Introduction
The spring framework in Java is used for building enterprise-class applications. This framework has extensive support for building REST-based web services. There are both client-side and server-side libraries available for this. The web applications that leverage the power of the Spring framework can implement REST services in a much faster way. This article will discuss the client-side REST implementation using the Spring framework.
The Spring REST Client
The client-side implementation of Spring REST will enable a client application to receive web service responses from the server. It also provides a toolset required for building and sending REST API requests from the client application. The main class that enabled the client to use the REST API with Spring was the RestTemplate class. But from the latest version of Spring, ie. Spring 5.0 onwards this class has been deprecated and a new class called WebClient got introduced. It’s a modern alternative to the RestTemplate class. Let us have a detailed look at the WebClient class.
WebClient Class
From Spring 5.0 onwards the WebClient class will be the gateway of all the web requests and responses in a web application. The advantages of this implementation over its predecessor RestTemplate class are:
(a) It is non-blocking in nature.
(b)It has a singleton implementation
(c) It is reactive in nature.
The WebClient class like its predecessor works over protocol HTTP/1.1. While working with the WebClient class the main steps we need to know are the following:
1. Creating an instance.
2. Building a client request.
3. Handling the request & response.
Let us get into the details of these processes.
- Creating an instance
The simplest way to create an instance is as shown below:
WebClient testClient = WebClient.create();
We can also create the instance by providing a URI:
WebClient testClient = WebClient.create(“http://exampleserver.com/8000”);
The most advanced way of creating an instance is by providing all the required details for the class. The below given code snippet is an example for that.
WebClient testClient = WebClient
.builder()
.baseUrl(“http://exampleserver:8000”)
.defaultCookie(“key”, “value”)
.defaultUriVariables(Collections.singletonMap(“url”, “http://exampleserver:8000“))
.defaultHeader(HttpHeaders.CONTENT_TYPE,
MediaType.APPLICATION_JSON_VALUE)
.build();
|
- Building a client request
Once we are ready with an instance, the next step is to create a REST API request from the client. One of the main parts of the request is the type of request that we are sending. We must decide the HTTP methods GET, POST, UPDATE & DELETE that we are going to use while sending a request. The following code snippet will do that:
WebClient.UriSpec testRequest = testRequest.method(HttpMethod.POST);
The next step is to provide a target URL which can be done as given below:
WebClient.RequestBodySpec testURI = testClient
.method(HttpMethod.POST)
.uri(“/resource”);
|
After this step, we have to provide the other details such as content-type, header details, etc. That can be set as follows:
WebClient.RequestBodySpec testURI = testClient
.method(HttpMethod.POST)
.uri(“/resources”).body( BodyInserters.fromPublisher( Mono.just(“information”)),
String.class);
|
Once we are done with setting the body of the request, the WebClient will send the request to the target URI which will invoke the server-side API function.
- Handling the request & response
There are two ways we can send and receive the information using WebClient. One is using the exchange() method and the other one is using the retrieve() method.
The main differences between these two methods are that the first method will return HTTP information like cookies, headers, etc along with the response body while the second method will only return the response body. A sample request & response code will look as follows:
Class testClass = testClient.post()
.uri(“/resources”)
.body(Mono.just(), String.class)
.header(HttpHeaders.CONTENT_TYPE, MediaType.APPLICATION_JSON_VALUE)
.retrieve()
.bodyToMono(String.class);
|
Here the request is a HTTP POST and the response will be returned as a response body which will be parsed by the bodyToMono() function. If there is a 4xx or 5xx response, this method will throw a WebClientException.
Summary
Handling requests and responses at the client side are handled in the Spring framework using the WebClient implementation. This is the new standard for handling client web requests and responses after Spring 5.0. There is a rich set of functionalities that makes this implementation an efficient and easier one.
Share