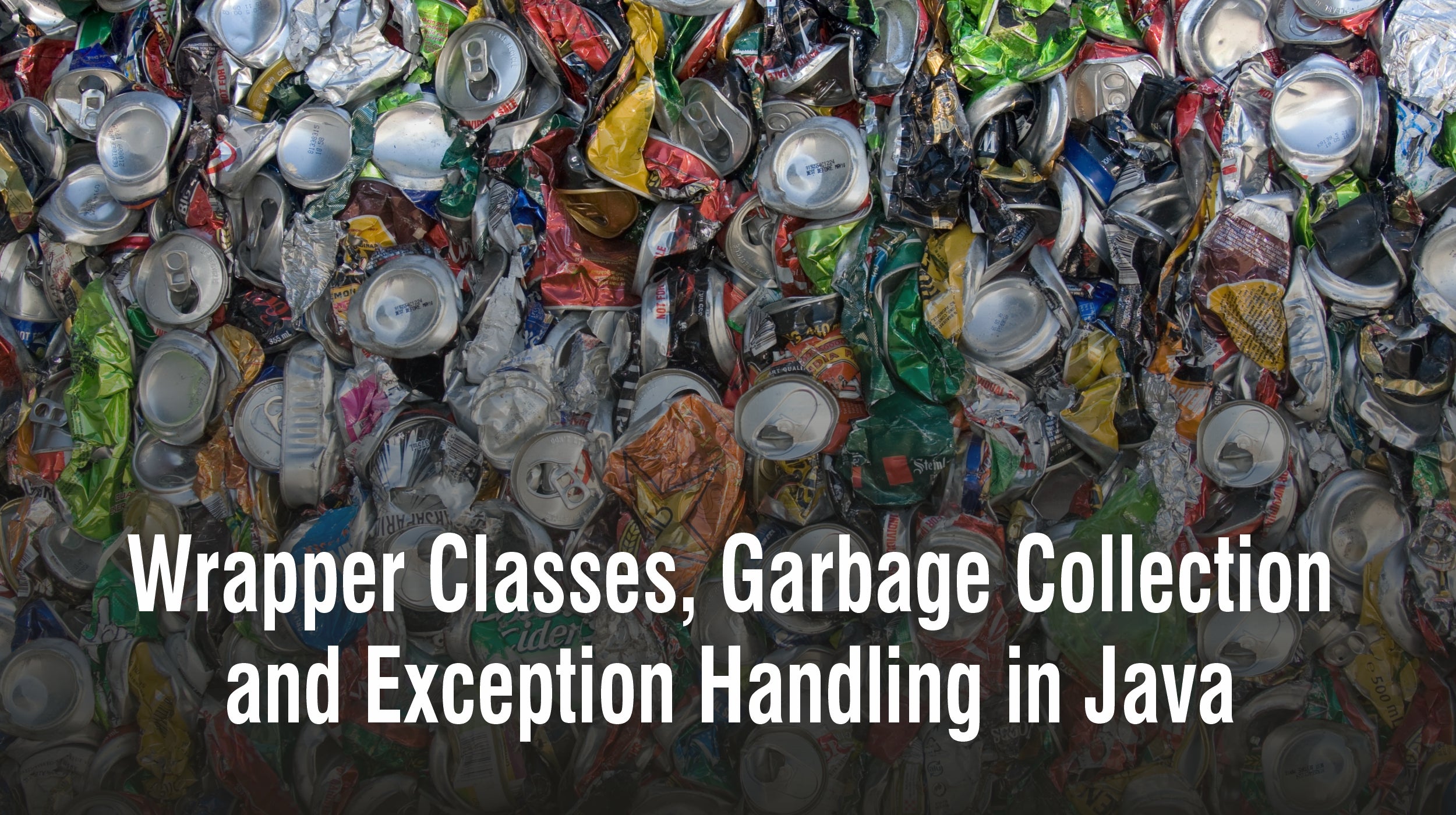
Wrapper Classes, Garbage Collection and Exception Handling in Java
Introduction
In this blog, we will look at some features of wrapper classes and how garbage collection works in Java.Java is an Object-oriented language. The primitive data types in Java are not objects. Sometimes, we will need object equivalents of the primitive types. An example is when we use collections. It contains only certain objects, not primitive types.To solve such issues, Java introduced Wrapper class that wraps primitive types into objects and each primitive has corresponding wrapper class.
Garbage collection is the process of freeing memory allocated to an object that is no longer used. During run-time, when objects are created, the Java virtual machine (JVM) allocates some memory to hold the object. The JVM uses the Mark and sweep algorithm internally for garbage collection.
Wrapper Classes:
As the name suggests, wrap means to cover something, and wrapper class in Java does the same. Wrapper classes in Java are objects encapsulating Java primitive data types.Each primitive data type has a corresponding wrapper in Java, as shown below:
Primitive Data Type boolean byte short char int long float double |
Wrapper Class Boolean Byte Short Char Int Long Float Double |
Since all these classes are part of java.lang.package we don’t need to import them explicitly.
But if you are wondering about its use case, you must know that in Java, all generic classes only work with objects and they do not support primitives. So when we have to work with primitive data types, we have to convert them into wrapper objects. That’s where wrapper classes come in handy.
This conversion can be done by either using a constructor or by using the static factory methods of a wrapper class. The example below shows how to convert an int value to Integer object in Java:
int intValue =1;
Integer object = new Integer.valueOf( intValue); |
Here, the valueOf() method will return an object with specified intValue.
Similarly, all other conversions can be done with corresponding wrapper classes.
Garbage Collection:
It is a memory management technique that the Java language has implemented by scanning heap memory (where Java objects are created) for unused objects and deleting them to free up the heap memory space.Garbage Collection is an automatic process that each JVM implements through threads called Garbage Collectors to eliminate any memory leaks. There are four types of garbage collectors in Java namely Serial, Parallel, CMS & G1 Garbage Collectors depending upon which part of heap memory you are doing the garbage collection on. We can make use of them based on our requirements.
The basic process of garbage collection is to first identify and mark the unreferenced objects that are ready. The next step is to delete the marked objects. Sometimes, memory compaction is performed to arrange remaining objects in a contiguous block at the start of the heap. Before the deletion of an object, the garbage collection thread invokes the finalize() method of that object that allows all types of cleanup.
The recommended way of calling a garbage collector programmatically is given below:
public static void gc() {
Runtime.getRuntime().gc();
} |
One important thing to note here is that Java is non-deterministic in garbage collection, i.e., there is no way to predict when it will occur at run-time. JVM will trigger the garbage collection automatically based on the heap size. We can include some hints for garbage collection in JVM using System.gc() or Runtime.gc() methods. But we can not force the garbage collection process.
Exception Handling:
An exception is an event that disrupts the normal execution of a program. Java uses exceptions to handle errors and other abnormal events during the execution of the program. The master class for this is class ’throwable’ in Java.When we discuss exceptions, we must understand that they are different from Errors. Errors are impossible to recover while exceptions are recoverable. Errors only occur at run-time and are caused by the Java run time environment, while exceptions can occur at compile time as well and the cause is the application itself, not the Java compiler.
In Java, exceptions that occur at compile time are called checked exceptions and run-time exceptions are called unchecked exceptions.
A basic example of an exception is shown below:
class SampleException{
public static void main(String args[]){
try {
//code that raise exception
}
catch (Exception e) {
// rest of the program }
}
} |
There are many built-in exceptions in Java.Users can also define their exceptions by extending the Exception class.
A new exception is thrown using the ‘throw’ or ‘throws’ keywords. If there is only one exception to throw we use the throw keyword:
public static void findRecord() throws IOException {
throw new IOException(“Unable to find record”);
} |
The main difference between throw & throws are:
Throw
|
Throws
|
An exception is handled using a statement in Java.The statements for monitoring errors are put within the try block. The code that needs to be executed in case an exception occurs should be placed within the catch block. In the finally block, you need to put in code that is executed irrespective of whether an exception is thrown or not.
- try : A try block is where you will put the code that may raise exceptions.
- catch: The catch block is used to handle a given exception.
- finally: The keyword ‘finally’ is used to define the code block that must be executed irrespective of the occurrence of an exception.
A sample code would be:
class ExceptionSample{
public static void main(String args[]){
try{
int intData= 0/3;
System.out.println(intData);
}
catch(NullPointerException e){
throw new NullPointerException(“There was an error in the calculation.”);
}
finally {System.out.println(“ We have completed the exception handling”);}
//remaining code
}
} |
End note:
In this blog we have studied wrapper classes and the necessity of it. We also looked at garbage collection and how it functions, as well as its types and the part of JVM in which it is stored. We briefly explored the process of Exception handling and its statement, which handles exceptional errors in the code.
Get one step closer to your dream job!
Prepare for your Java programming interview with Core Java Questions You’ll Most Likely Be Asked. This book has a whopping 290 technical interview questions on Java Collections: List, Queues, Stacks, and Declarations and access control in Java, Exceptional Handling, Java Operators, Java 8 and Java 9, etc. and 77 behavioral interview questions crafted by industry experts and interviewers from various interview panels.
We’ve updated our blogs to reflect the latest changes in Java technologies.
This blog was previously uploaded on March 26th, 2020 and has been updated on January 11th, 2022.
Share