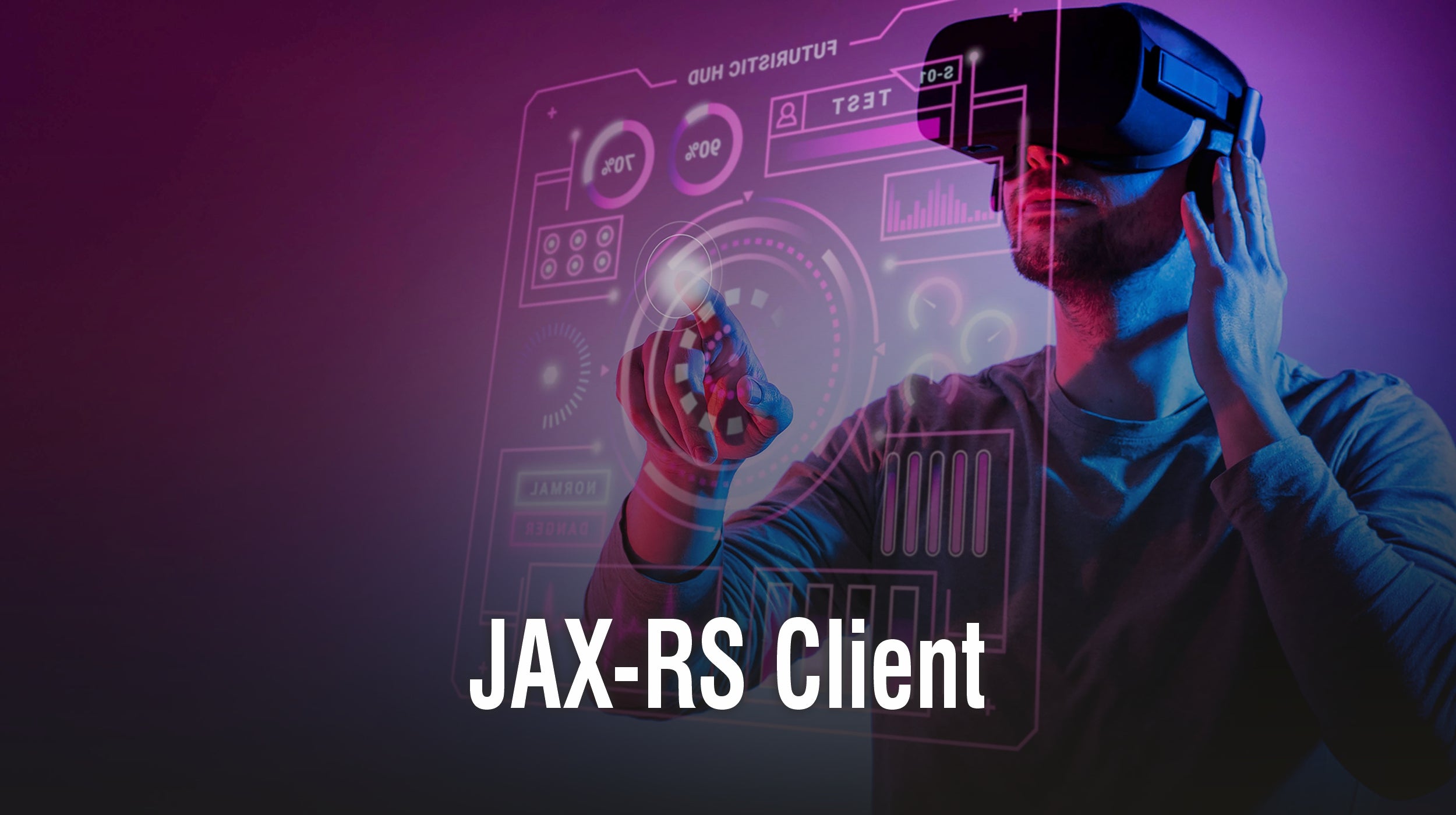
JAX-RS Client
by Vibrant Publishers
Introduction
Resources form the basic building block of any REST service. These resources are accessed using the REST client libraries. JAX-RS client is such an implementation. This library makes it easy for any Java programmer to access any REST resources over the internet.
JAX-RS Client
The first version of JAX-RS ie. JAX-RS 1.1 does not had any client implementation. It is from JAX-RS 2.0 onwards it started supporting REST clients.
The JAX-RS client API can be found under the javax.ws.rs.Client package. Classes under this package provide high-level APIs for accessing any REST resources, not just JAX-RS services.
Let us have a look into the basic operations of JAX-RS Client API.
Creating a basic client request
Before defining a client request, we have to set certain things in place. They are:
- Obtain an instance of javax.ws.rs.Client class.
- Configure the client with a resource target.
- Create a request.
- Invoke the client request.
Let’s have a look into these steps by considering the following code snippet:
Client apiClient = ClientBuilder.newClient();
String URI = “http://foodchain.com/api/foodmenu”;
String foodMenu = client.target(URI).request(MediaType.JSON).get(String.class);
client.close(); |
Here we first created the client instance by declaring the client variable using the newClient() method.
Client apiClient = ClientBuilder.newClient();
After this step, we are chaining a set of methods with the client instance that will return the API resource to the string variable foodMenu. There are three steps involved in this process.
- Setting the target by apiClient.target(URI). This is the remote location where the resource is located.
- Building a REST request entity using the apiClient.request(Mediatype.JSON) statement. Here we also set the media type of the entity.
- Invoking the HTTP GET request with apiClient.target(URI).request(MediaType.JSON).get(String.class) statement. Also, the return type of the entity is set into String type.
Once the client API successfully receives the response, we will close the client connection with the apiClient.close() statement.
We have now seen a simple JAX-RS client API call in action. Now let us look into some of the extended use cases of JAX-RS client library.
Setting multiple client targets
We used the client.target() method in the previous example to set the target URI. We can also use the javax.ws.rs.client.WebTarget class for the same purpose. This will help us in handling REST resources where multiple targets are involved.
In the given example, we are setting a base target “http://restapi.com/assignments” using the WebTarget class. The base target is used for building several resource targets that will point to different API services provided by a given RESTful resource.
Client apiClient = ClientBuilder.newClient();
WebTarget base = apiClient.target(“http://restapi.com/assignments”);
// WebTarget at http://restapi.com/assignments/read
WebTarget read = base.path(“read”);
// WebTarget at http://restapi.com/assignments/write
WebTarget write = base.path(“write”);
|
Invoking the WebTarget.path() method with a path parameter creates a new WebTarget instance by appending it with the base URI.
Invoking the request
The JAX-RS client can invoke every available REST request method. This is accomplished by calling the WebTarget.request() method and passing the relevant media type value. This will provide an instance of javax.ws.rs.client.Invocation.Builder. This helper object will provide HTTP methods for the client API request.
In the first example, apiClient.target(URI).request(MediaType.JSON).get(String.class) we had invoked the HTTP GET request and the same process stands for POST(), DELETE(), PUT() methods also.
Summary
JAX-RS client API that got introduced in version 2.0 is one of the best implementations of the REST client library. It’s quite easy to make client API calls using this library. We can use this library in Java for accessing any REST resource over the web.
Share