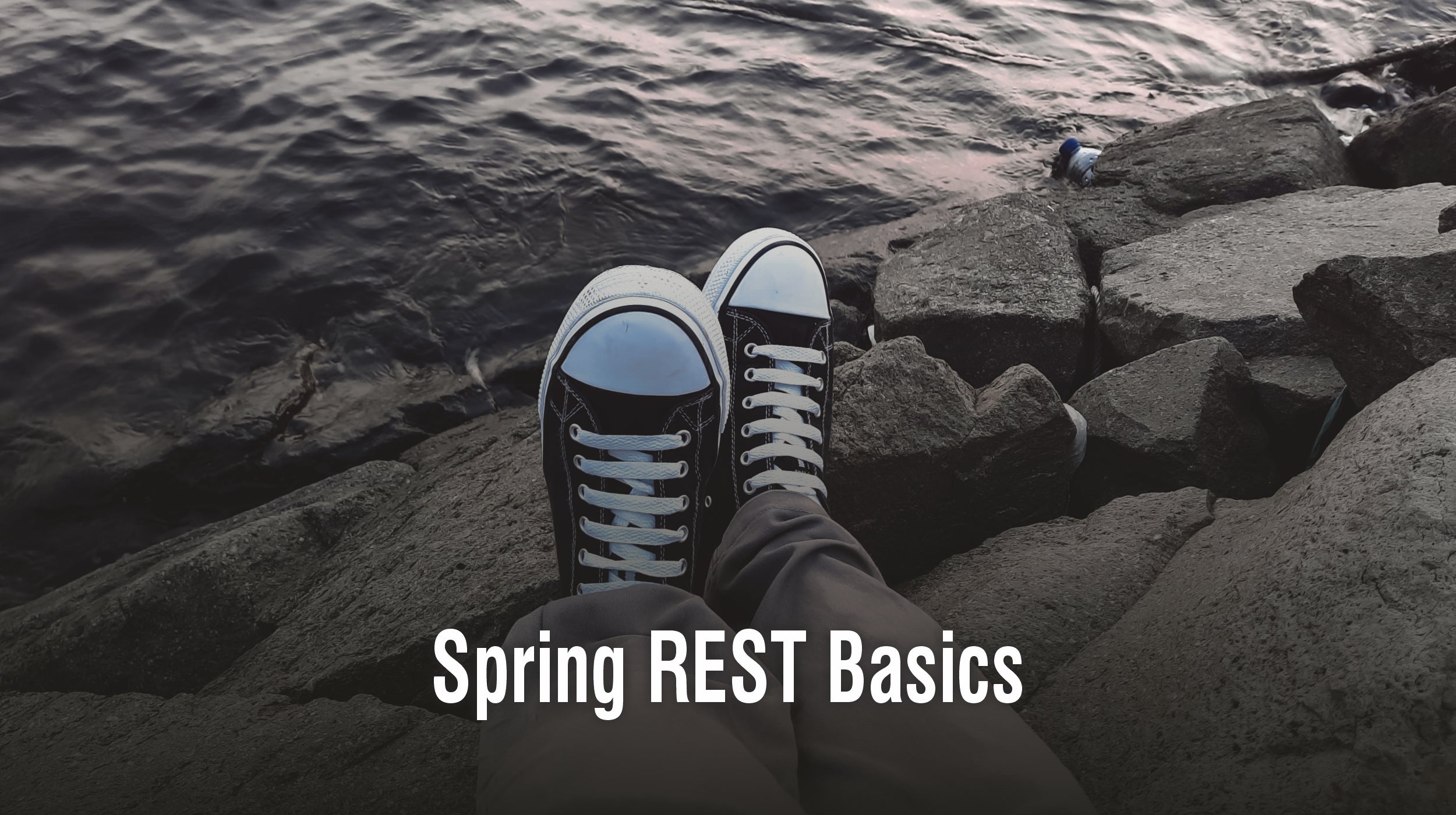
Spring REST Basics
Introduction
Spring is a popular Java application framework. The spring framework is based on a principle called dependency injection which allows developers to build completely decoupled systems. This framework supports all Java features and also there are extensions for this framework that allows us to build web applications leveraging the JavaEE platform. In this article, we are exploring the basics of REST implementation in the Spring framework.
Spring and REST
The REST API specification in the Java world is known as JAX-RS. Spring framework has included this specification and is available under the Spring Web package. The spring framework has support for other Java REST implementations like Jersey as well. Within the Spring framework, there are two ways we can implement the REST web services.
- Spring MVC: Uses the traditional model-view-control method. Configuration heavy. The below given diagram will provide an idea about the MVC model. This is a typical multi layered approach for handling REST requests and responses.
- SpringBoot: Leverages the HTTP message converters. More modern (Spring 3.0) and easy to implement.
We will be using SpringBoot for developing our REST applications using Spring.
Setting up a Spring REST application
When we develop applications using the Spring framework, it’s a good practice to use the Spring Initializr page. This will help you define the project with all dependencies and generate your project configuration file that will save a lot of your project setup time.
All the REST applications using SpringBoot will have a SpringWeb dependency that we will choose while creating the project using the Spring Initializr. There are two build systems available here. Gradle and Maven. Gradle will generate a build configuration file namely build.gradle and Maven will use the pom.xml file for build configurations. All your dependencies and external libraries must be included in this file. We will be using the Gradle build system here.
In the configuration, first thing you have to include is the Spring Boot Starter Web dependency. In Gradle it will look like the following:
#compile(‘org.springframework.boot:spring-boot-starter-web’
The complete configuration file for the Gradle build will look like something as given below:
buildscript { ext { springBootVersion = ‘2.2.6.RELEASE’ } repositories { mavenCentral() }
Dependencies{ classpath( “org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}” ) } } apply plugin:’org.springframework.boot’ apply plugin:’java’ apply plugin:’eclipse’ group = ‘com.example’ version = ‘0.0.1-SNAPSHOT’ sourceCompatibility = 1.8 repositories { mavenCentral() } Dependencies{ compile( ‘org.springframework.boot:spring-boot-starter-web’ ) testCompile( ‘org.springframework.boot:spring-boot-starter-test’ ) } |
Basic Annotations
Before we start building the REST application using Spring, let us understand some of the annotations that we will be using in the process.
Rest Controller
The @RestController annotation is used for including the main artifact of the RESTful API in our code. This is also responsible for providing JSON, XML responses and any other custom responses.
Request Mapping
@RequestMapping annotation is used for defining the URI to access the endpoints. We will use a request method for consuming and producing resources. The default request method is GET.
Path Variable
When we need to set a custom request URI. The annotation @PathVariable will be specific to each resource and can have dynamic values.
Request Body
When we send a request the content type of the request is mentioned using @RequestBody annotation.
Request Parameter
The @RequestParam annotation is used for extracting parameters from the request URL. This parameter must be included while we send a request.
Summary
The Spring framework is an enterprise application development framework for Java. The JAX-RS specifications for REST web services are well integrated with Spring and are available with many rich features. The SpringBoot package is the modern implementation in Spring that can be used for creating REST applications with ease and flexibility.
Share