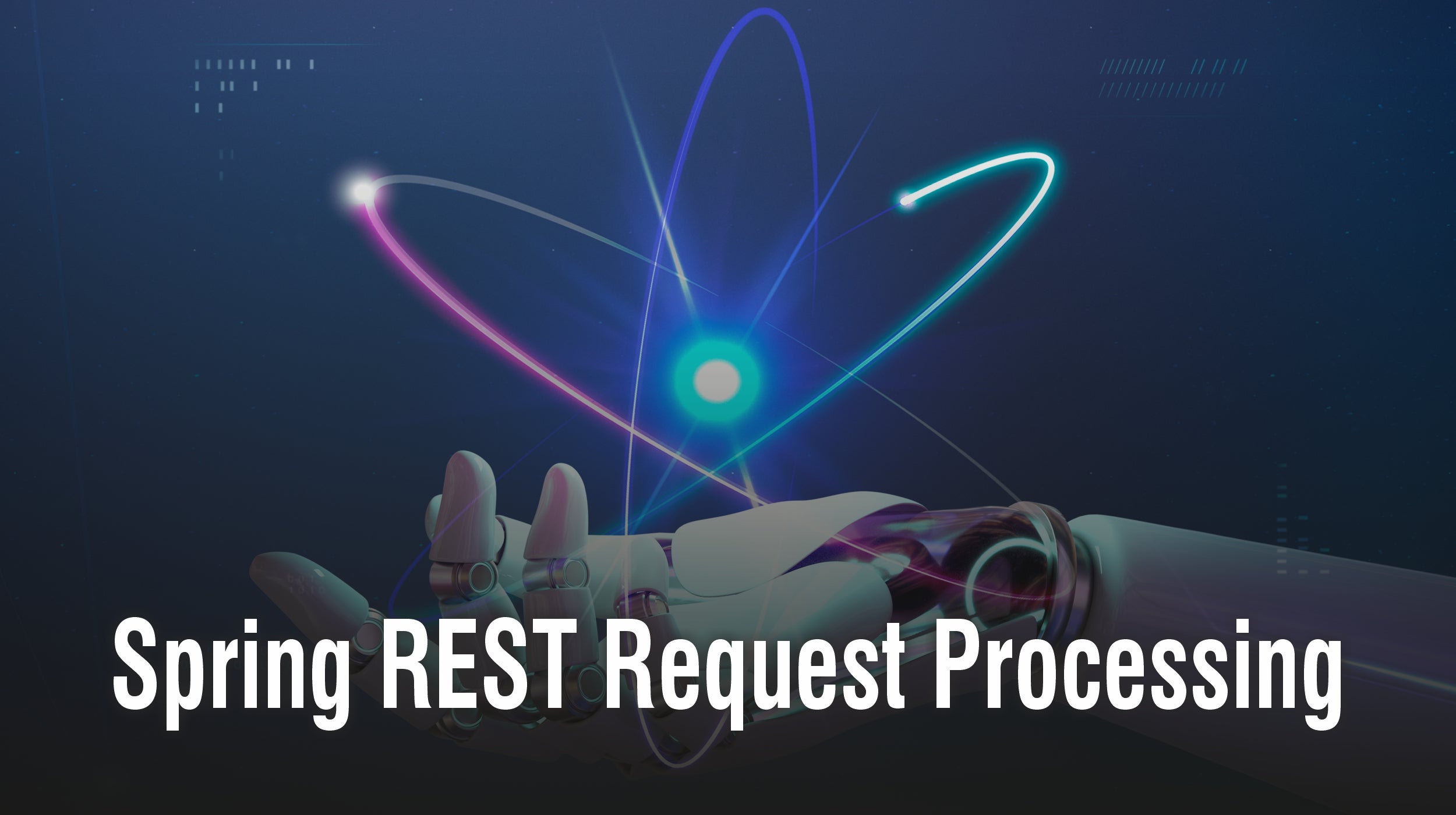
Spring REST Request Processing
Introduction
Developing REST APIs and web services using Spring Boot makes the process very easy and helpful for the developer. It takes out a lot of overheads and helps us to only focus on the core business logic. The rest of the configurations and setup processes are taken care of by the framework itself. In this article, we will focus on the request processing part of a REST API using Spring Boot.
The flow of an API Request in Spring
When we create a microservice in Spring the main control flow of an incoming request will as shown below:
The role of annotations
Some of the main annotations for processing a request using spring are as follows:
- @RestController: This is the heart of any Spring web service. There must be a class declared with this annotation. It will make the class discoverable during classpath scanning.
- @RequestMapping: The resource methods that are annotated with the above annotation will be exposed as HTTP endpoints. When an API request matches a given @RequestMapping annotation, the resource method will be executed to serve the incoming request.
The following code snippet will give you an idea of using these annotations:
The request mapping process
@RestController
@RequestMapping(“/employee”)
public class EmployeeController {
@Autowired
EmployeeDetails employeeDetails
@RequestMapping(method = RequestMethod.GET)
public List findEmployee()
{
return employeeDetails.findEmployee();
}
}
|
When we prepare our classes and resource methods with the given annotations, the Spring Boot takes care of the rest of the processing.
Let us consider a sample incoming request as given below:
Request: http://localhost:8080/employee
Accept: application/JSON
When this request reaches the server container, the spring boot will handle this request using a DispatcherServlet. The configuration and initialization of this servlet will be already done by the Spring Boot during the project creation time itself.
The Dispatcher Servlet
The DispatcherServlet will be the front end controller through which all the requests will be passing through. There are a few steps that the DispatcherServlet will be doing for us to process the request. They are:
- Intercepting the incoming request to identify the resource.
- Finding a suitable handler for the given request. This is done by checking the Path parameter, HTTP method, Query parameter & the Request header.
- Get the matching controller method for the incoming request. This is possible due to the mapping registry prepared by the spring framework by looking up @RequestMapping annotations.
- Execute the controller method to process the incoming resource.
We can understand the process from the following diagram:
Summary
Understanding the processes involved in REST request handling by the spring ecosystem is important. This will help us to code efficiently and also in doing better troubleshooting.
Share